C Exercises: Create enumerated data type for 7 days and display their values in integer constants
Enumerate and display integer values for the days of the week
Write a C program to create enumerated data types for 7 days and display their values in integer constants.
Pictorial Presentation:
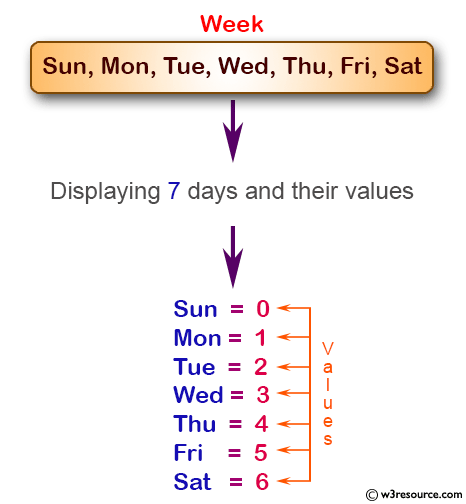
Sample Solution:
C Code:
#include <stdio.h>
int main() {
// Define an enumeration type 'week'
enum week {Sun, Mon, Tue, Wed, Thu, Fri, Sat};
// Print the values of the days using the enumeration constants
printf("Sun = %d", Sun);
printf("\nMon = %d", Mon);
printf("\nTue = %d", Tue);
printf("\nWed = %d", Wed);
printf("\nThu = %d", Thu);
printf("\nFri = %d", Fri);
printf("\nSat = %d", Sat);
return 0;
}
Sample Output:
Sun = 0 Mon = 1 Tue = 2 Wed = 3 Thu = 4 Fri = 5 Sat = 6
Flowchart:
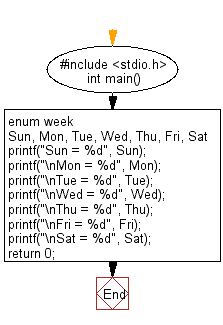
For more Practice: Solve these Related Problems:
- Write a C program to create an enum for the days of the week and print each day with its integer value using a loop.
- Write a C program to map the days of the week to integer values and display them in reverse order.
- Write a C program to generate an enum for days and allow the user to select a day for display.
- Write a C program to create an enum for days and print only the weekend days with their integer values.
C programming Code Editor:
Previous:Write a C program to display sum of series 1 + 1/2 + 1/3 + ………. + 1/n.
Next: Write a C program that accepts a real number x and prints out the corresponding value of sin(1/x) using 4-decimal places.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.