Validate a password (1234 as correct)
Validate a password (1234 as correct)
Write a C program to read a password until it is valid. For wrong password print "Incorrect password" and for correct password print, "Correct password" and quit the program. The correct password is 1234.
C Code:
#include <stdio.h>
int main() {
int pass, x = 10; // Initialize variables for password and loop control
while (x != 0) {
printf("\nInput the password (numeric characters only): ");
scanf("%d", &pass); // Read the password input
if (pass == 1234) {
printf("Correct password"); // If the password is correct, print a success message
x = 0; // Set x to 0 to exit the loop
} else {
printf("Wrong password, try another"); // If the password is incorrect, prompt for another attempt
}
printf("\n");
}
return 0;
}
Sample Output:
Input the password: 1234 Correct password
Flowchart:
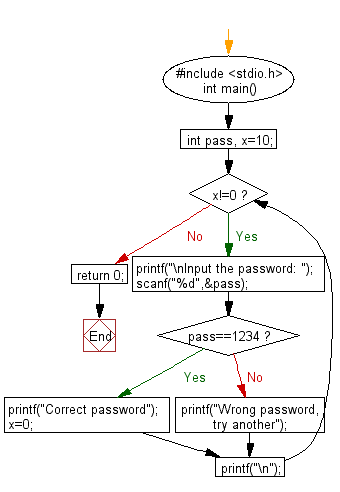
For more Practice: Solve these Related Problems:
- Write a C program to validate a password with a maximum of three attempts before locking the user out.
- Write a C program to check a password ensuring it contains at least one uppercase letter, one lowercase letter, and one digit.
- Write a C program to validate a password and lock the system after a specified number of incorrect attempts.
- Write a C program to validate a password based on case sensitivity and a minimum length requirement.
C Programming Code Editor:
Previous: Write a C program to check if two numbers in a pair is in ascending order or descending order.
Next: Write a C program to read the coordinate(x, y) (in Cartesian system) and find the quadrant to which it belongs (Quadrant -I, Quadrant -II, Quadrant -III, Quadrant -IV).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.