Count positives and calculate their average from 5 inputs
C Practice Exercise
Write a C program that reads 5 numbers, counts the number of positive numbers, and prints out the average of all positive values.
Pictorial Presentation:
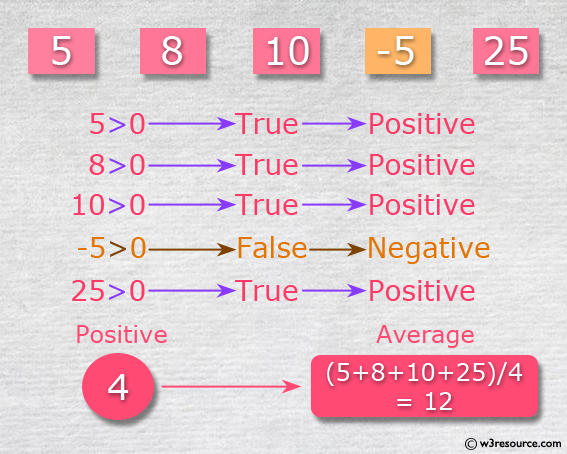
C Code:
#include <stdio.h>
int main() {
float numbers[5], total=0, avg; // Declare an array to store 5 numbers, and variables for total and average
int j, pctr=0; // Declare variables for loop counter and positive count
// Prompt user for five numbers and store them in the array
printf("\nInput the first number: ");
scanf("%f", &numbers[0]);
printf("\nInput the second number: ");
scanf("%f", &numbers[1]);
printf("\nInput the third number: ");
scanf("%f", &numbers[2]);
printf("\nInput the fourth number: ");
scanf("%f", &numbers[3]);
printf("\nInput the fifth number: ");
scanf("%f", &numbers[4]);
for(j = 0; j < 5; j++) {
if(numbers[j] > 0) // Check if the number is positive
{
pctr++; // Increment positive count
total += numbers[j]; // Add positive number to total
}
}
avg = total/pctr; // Calculate average of positive numbers
// Print the counts of positive numbers and the average
printf("\nNumber of positive numbers: %d", pctr);
printf("\nAverage value of the said positive numbers: %.2f", avg);
printf("\n");
return 0;
}
Sample Output:
Input the first number: 5 Input the second number: 8 Input the third number: 10 Input the fourth number: -5 Input the fifth number: 25 Number of positive numbers: 4 Average value of the said positive numbers: 12.00
Flowchart:
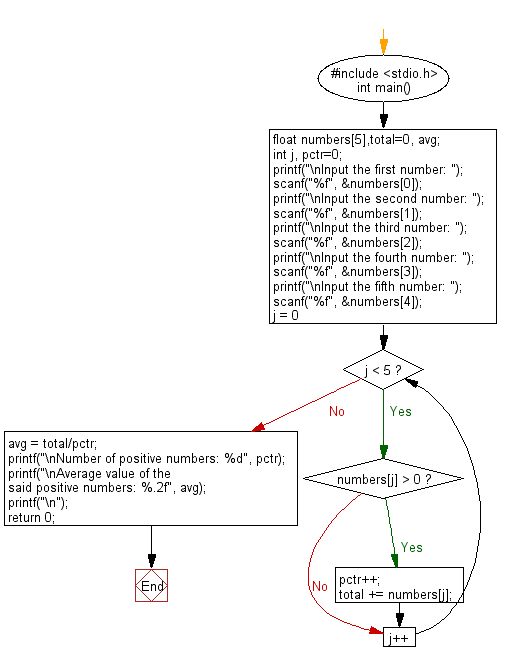
For more Practice: Solve these Related Problems:
- Write a C program to count negative numbers and calculate their average from five inputs.
- Write a C program to calculate the average of numbers that are above a specified threshold from a set of inputs.
- Write a C program to count and calculate the average of even numbers from a series of five integers.
- Write a C program to count numbers meeting two conditions (e.g., positive and even) and then compute their average.
C Programming Code Editor:
Previous: Write a C program that read 5 numbers and counts the number of positive numbers and negative numbers.
Next: Write a C program that read 5 numbers and sum of all odd values between them.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics