Print all even numbers between 1 and 50
Print all even numbers between 1 and 50
Write a C program that prints all even numbers between 1 and 50 (inclusive).
Pictorial Presentation:
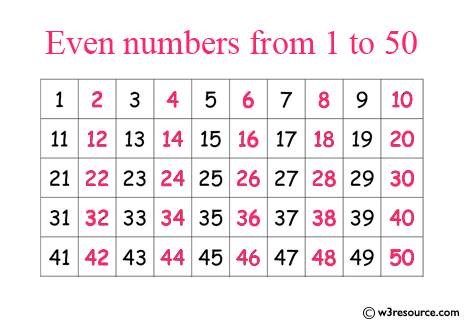
C Code:
#include <stdio.h>
int main() {
int i; // Declare variable for loop counter
printf("Even numbers between 1 to 50 (inclusive):\n");
for (i = 1; i <= 50; i++)
{
if(i%2 == 0) // Check if 'i' is even
{
printf("%d ", i); // Print 'i' if it's even
}
}
return 0;
}
Sample Output:
Even numbers between 1 to 50 (inclusive): 2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50
Flowchart:
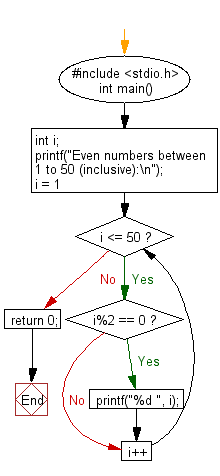
For more Practice: Solve these Related Problems:
- Write a C program to print all odd numbers between 1 and 50 (inclusive).
- Write a C program to print all prime numbers between 1 and 50.
- Write a C program to display Fibonacci numbers up to a maximum value of 50.
- Write a C program to print numbers between 1 and 50 that are divisible by 3.
C Programming Code Editor:
Previous: Write a C program that reads an integer between 1 and 12 and print the month of the year in English.
Next: Write a C program that read 5 numbers and counts the number of positive numbers and negative numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.