Convert days to years, months, days
C Practice Exercise
Write a C program to convert a given integer (in days) to years, months and days, assuming that all months have 30 days and all years have 365 days.
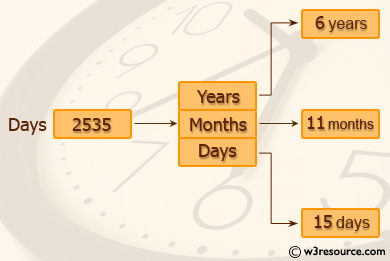
C Code:
#include <stdio.h>
int main() {
int ndays, y, m, d; // Declare variables for number of days, years, months, and days
// Prompt user for input number of days and store in 'ndays'
printf("Input no. of days: ");
scanf("%d", &ndays);
// Calculate years, months, and remaining days
y = (int) ndays/365;
ndays = ndays-(365*y);
m = (int)ndays/30;
d = (int)ndays-(m*30);
// Print the result
printf(" %d Year(s) \n %d Month(s) \n %d Day(s)", y, m, d);
return 0;
}
Sample Output:
Input no. of days: 2535 6 Year(s) 11 Month(s) 15 Day(s)
Flowchart:
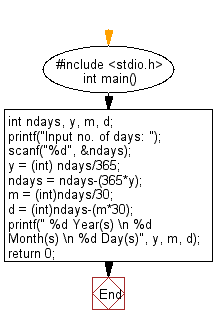
For more Practice: Solve these Related Problems:
- Write a C program to convert a given number of days into weeks and remaining days.
- Write a C program to convert days into years, weeks, and days, accounting for leap years in the calculation.
- Write a C program to convert a total number of days into years, months (assuming variable month lengths), and days.
- Write a C program to perform a detailed time breakdown for a large number of days into years, months, weeks, and days.
C Programming Code Editor:
Previous: Write a C program to convert a given integer (in seconds) to hours, minutes and seconds.
Next: Write a C program that accepts 4 integers p, q, r, s from the user where q, r and s are positive and p is even. If q is greater than r and s is greater than p and if the sum of r and s is greater than the sum of p and q print "Correct values", otherwise print "Wrong values".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.