C Exercises: Accepts three integers and find the maximum
C Practice Exercise
Write a C program that accepts three integers and finds the maximum of three.
Pictorial Presentation:
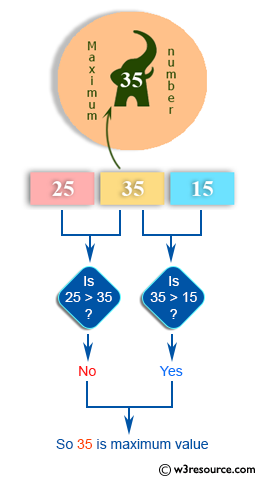
C Code:
#include <stdio.h>
#include <stdlib.h>
int main()
{
int x, y, z, result, max; // Declare variables
// Prompt user for the first integer and store in 'x'
printf("\nInput the first integer: ");
scanf("%d", &x);
// Prompt user for the second integer and store in 'y'
printf("\nInput the second integer: ");
scanf("%d", &y);
// Prompt user for the third integer and store in 'z'
printf("\nInput the third integer: ");
scanf("%d", &z);
// Calculate the result
result = (x + y + abs(x - y)) / 2;
// Calculate the maximum value
max = (result + z + abs(result - z)) / 2;
// Print the maximum value
printf("\nMaximum value of three integers: %d", max);
printf("\n");
return 0;
}
Sample Output:
Input the first integer: 25 Input the second integer: 35 Input the third integer: 15 Maximum value of three integers: 35
Flowchart:
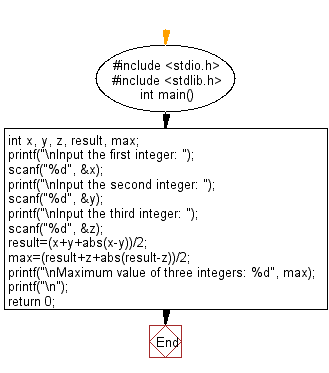
For more Practice: Solve these Related Problems:
- Write a C program to find the minimum value among three integers provided by the user.
- Write a C program to find both the maximum and minimum values among three integers.
- Write a C program to find the maximum value from a list of integers entered by the user.
- Write a C program to determine the second largest number among three given integers.
C Programming Code Editor:
Previous: Write a C program that accepts an employee's ID, total worked hours of a month and the amount he received per hour. Print the employee's ID and salary (with two decimal places) of a particular month.
Next: Write a C program to calculate a bike’s average consumption from the given total distance (integer value) traveled (in km) and spent fuel (in liters, float number – 2 decimal point).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.