C Exercises: Sum of n even numbers starting from m
Compute sum of nnn even numbers starting from mmm
Write a C program that reads two integers m, n and computes the sum of n even numbers starting from m.
Sample Solution:
C Code:
Sample Output:
Input two integes (m, n): 20 60 Sum of 60 even numbers starting from 20: 4740
Flowchart:
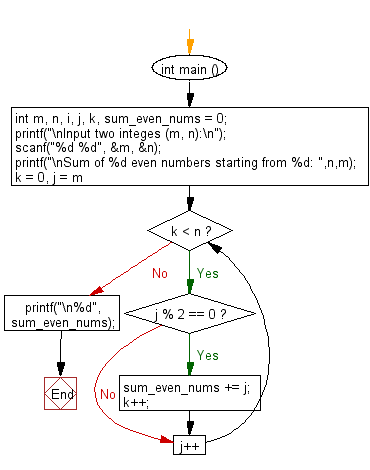
For more Practice: Solve these Related Problems:
- Write a C program to calculate the sum of n consecutive even numbers starting from m using a for-loop.
- Write a C program to generate the sequence of even numbers starting at m and compute their sum iteratively.
- Write a C program to use a while-loop to accumulate the sum of n even numbers, starting from a user-defined m.
- Write a C program to compute the sum of even numbers in a range starting from m using arithmetic progression formulas.
C programming Code Editor:
Previous: Write a C program that reads an integer and find all the divisors of the said integer.
Next: Write a C program that reads two integers m, n and compute the sum of n odd numbers starting from m.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.