Print employee ID and monthly salary
C Practice Exercise
Write a C program that accepts an employee's ID, total worked hours in a month and the amount he received per hour. Print the ID and salary (with two decimal places) of the employee for a particular month.
C Code:
#include <stdio.h>
int main() {
char id[10]; // Variable to store employee ID (up to 10 characters)
int hour; // Variable to store working hours
double value, salary; // Variables for hourly salary and total salary
// Prompt user for employee ID
printf("Input the Employees ID(Max. 10 chars): ");
scanf("%s", &id);
// Prompt user for working hours
printf("\nInput the working hrs: ");
scanf("%d", &hour);
// Prompt user for hourly salary
printf("\nSalary amount/hr: ");
scanf("%lf", &value);
// Calculate total salary
salary = value * hour;
// Print employee ID and salary
printf("\nEmployees ID = %s\nSalary = U$ %.2lf\n", id, salary);
return 0;
}
Sample Output:
Input the Employees ID(Max. 10 chars): 0342 Input the working hrs: 8 Salary amount/hr: 15000 Employees ID = 0342 Salary = U$ 120000.00
Flowchart:
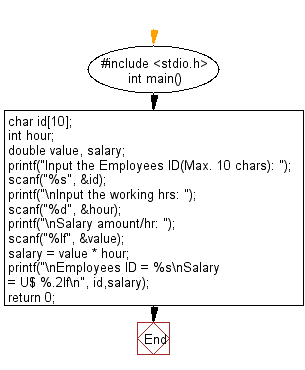
For more Practice: Solve these Related Problems:
- Write a C program to calculate an employee's gross salary including overtime pay, given worked hours and hourly rate.
- Write a C program to display employee ID and monthly salary in a formatted table for multiple employees.
- Write a C program to compute the monthly salary after deducting taxes and other allowances from the gross salary.
- Write a C program to print an employee's ID along with their salary formatted in different currency formats (e.g., USD, EUR).
C Programming Code Editor:
Previous: Write a C program that accepts two item’s weight (floating points' values ) and number of purchase (floating points' values) and calculate the average value of the items.
Next: Write a C program that accepts three integers and find the maximum of three.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.