C Exercises: Sum of all odd, even values between two integers
Sum odd and even numbers between two integers
Write a C program that reads two integer values and calculates the sum of all odd numbers between them.
Sample Solution:
C Code:
Sample Output:
Input the first integer number: 25 Input the second integer number (greater than first integer): 45 Sum of all odd values between 25 and 45: 385 Sum of all even values between 25 and 45: 350
Flowchart:
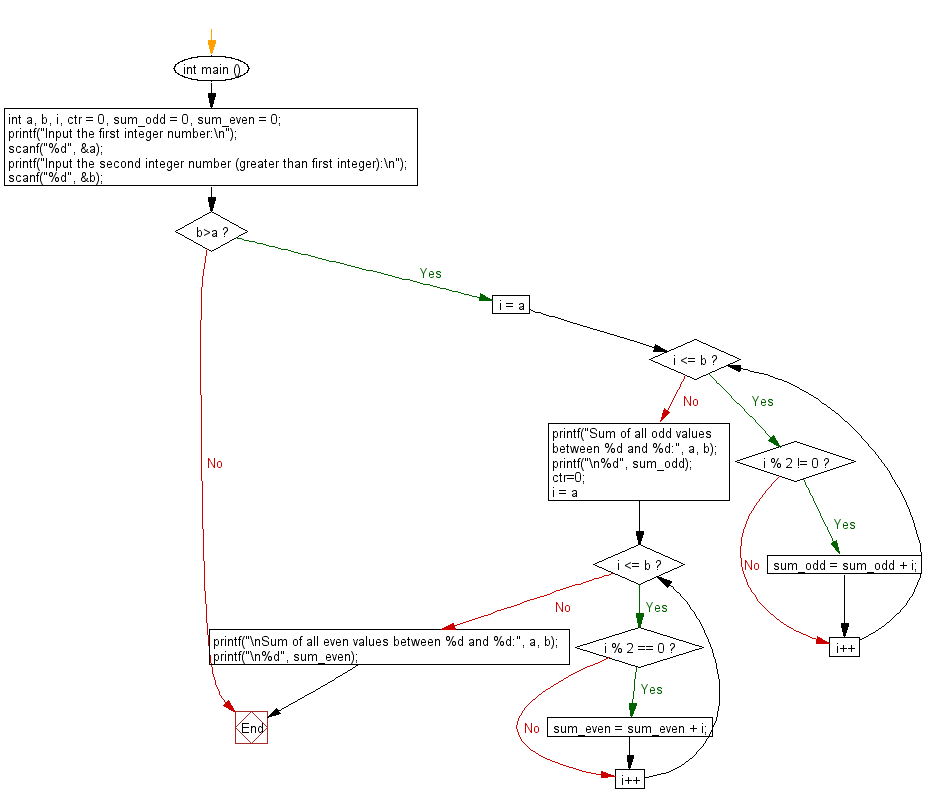
For more Practice: Solve these Related Problems:
- Write a C program to calculate the sum of odd and even numbers between two integers using separate loops.
- Write a C program that determines the range boundaries and sums odd and even numbers in one pass.
- Write a C program to compute and display both sums using a single loop with conditional checks for parity.
- Write a C program to sum odd and even numbers in a range using recursion to accumulate separate totals.
C programming Code Editor:
Previous: Write a C program that accepts an integer and print next ten consecutive odd and even numbers.
Next: Write a C program to find and print the square of each even and odd values between 1 and a given number (4 < n < 101).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.