Print name, DOB, and mobile number
C Practice Exercise
Write a C program to print your name, date of birth, and mobile number.
Pictorial Presentation:
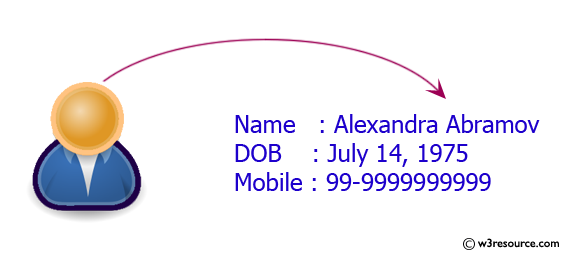
C Code:
#include <stdio.h>
int main()
{
// Print Name
printf("Name : Alexandra Abramov\n");
// Print Date of Birth
printf("DOB : July 14, 1975\n");
// Print Mobile Number
printf("Mobile : 99-9999999999\n");
// Indicate successful execution
return(0);
}
Explanation:
In the exercise above -
- #include <stdio.h>: This line includes the standard input-output library, which contains functions for reading and writing data to and from the console.
- int main(): This is the main function of the program, where execution begins. It returns an integer value, typically 0, to indicate successful execution.
- Inside the "main()" function, there are three printf statements. The "printf()" function is used to print formatted text to the console. Each printf statement prints a line of text with specific information:
- printf("Name : Alexandra Abramov\n"); prints "Name : Alexandra Abramov" followed by a newline character, which moves the cursor to the next line.
- printf("DOB : July 14, 1975\n"); prints "DOB : July 14, 1975" followed by a newline character.
- printf("Mobile : 99-9999999999\n"); prints "Mobile : 99-9999999999" followed by a newline character.
- return(0);: This line indicates the end of the main function and returns 0.
Sample Output:
Name : Alexandra Abramov DOB : July 14, 1975 Mobile : 99-9999999999
Flowchart:
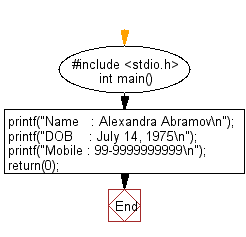
For more Practice: Solve these Related Problems:
- Write a C program to display your full name, date of birth, and mobile number with right-aligned fields using width specifiers.
- Write a C program to print your personal details where each field is padded with zeros to a fixed length.
- Write a C program to output your name, DOB, and mobile number with the name in uppercase and mobile number masked except last 4 digits.
- Write a C program to print your personal details in two columns, using formatted output and ensuring proper alignment.
C Programming Code Editor:
Previous: C Basic Declarations and Expressions Exercises Home
Next: Write a C program to print a block F using hash (#), where the F has a height of six characters and width of five and four characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.