C Exercises: Check if a given array of integers contains a 3 or a 5
58. Array Contains 3 or 5
Write a C program to check if a given array of integers contains a 3 or a 5.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
int arr_size;
// Declaration and initialization of an integer array 'array1'
int array1[] = {5, 5, 5, 5, 5};
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of the 'test' function for 'array1'
printf("%d",test(array1, arr_size));
// Declaration and initialization of an integer array 'array2'
int array2[] = { 1, 6, 8, 10};
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of the 'test' function for 'array2'
printf("\n%d",test(array2, arr_size));
// Declaration and initialization of an integer array 'array3'
int array3[] = {3, 3, 3, 5, 5, 5};
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of the 'test' function for 'array3'
printf("\n%d",test(array3, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
// Looping through the elements of the array
for (int i = 0; i < arr_size; i++)
{
// Checking if the current element is neither 3 nor 5
if (nums[i] != 3 && nums[i] != 5)
{
return 0; // If found, return 0 (false)
}
}
return 1; // If no other element is found, return 1 (true)
}
Sample Output:
1 0 1
Pictorial Presentation:
Flowchart:
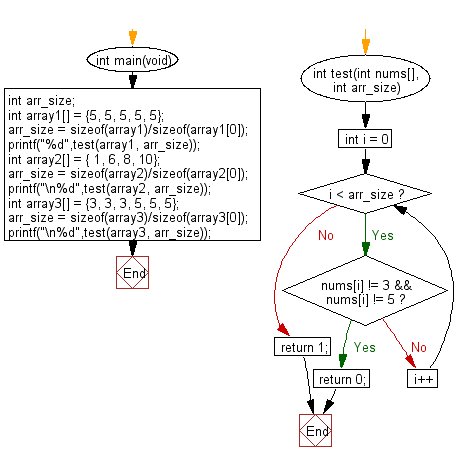
For more Practice: Solve these Related Problems:
- Write a C program to check if an array contains either 7 or 9.
- Write a C program to determine if an array contains at least one even or one odd number.
- Write a C program to verify if an array contains a 2 or a 4.
- Write a C program to check if an array contains either a negative or a positive number.
C Programming Code Editor:
Previous: Write a C program to check if the number of 3's is greater than the number of 5's.
Next: Write a C program to check if a given array of integers contains no 3 or a 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.