C Exercises: Check if the number of 3's is greater than the number of 5's
57. Compare Count of 3's and 5's
Write a C program to check if the number of 3's is greater than the number of 5's.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
int arr_size;
// Declaration and initialization of an integer array 'array1'
int array1[] = {1, 5, 6, 9, 3, 3};
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of the 'test' function for 'array1'
printf("%d",test(array1, arr_size));
// Declaration and initialization of an integer array 'array2'
int array2[] = {1, 5, 5, 5, 10, 17};
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of the 'test' function for 'array2'
printf("\n%d",test(array2, arr_size));
// Declaration and initialization of an integer array 'array3'
int array3[] = {1, 3, 3, 5, 5, 5};
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of the 'test' function for 'array3'
printf("\n%d",test(array3, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
int no_3 = 0, no_5 = 0;
// Looping through the elements of the array
for (int i = 0; i < arr_size; i++)
{
// Checking if the current element is equal to 3 or 5
if (nums[i] == 3)
{
no_3++; // Incrementing count of 3s
}
if (nums[i] == 5)
{
no_5++; // Incrementing count of 5s
}
}
// Comparing the counts of 3s and 5s, and returning the result
return no_3 > no_5;
}
Sample Output:
1 0 0
Pictorial Presentation:
Flowchart:
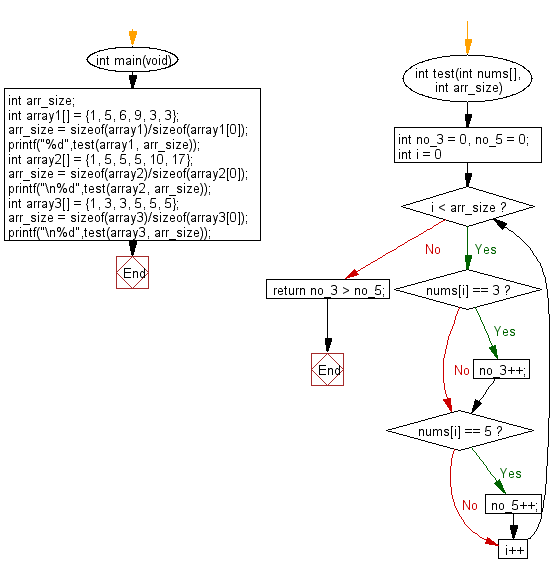
For more Practice: Solve these Related Problems:
- Write a C program to determine if the count of 2's is less than the count of 4's in an array.
- Write a C program to check if the frequency of a given digit is exactly twice that of another digit.
- Write a C program to verify if an array contains more even numbers than odd numbers.
- Write a C program to count the number of times a particular digit appears and compare it with another digit's frequency.
C Programming Code Editor:
Previous: Write a C program to check if the sum of all 5' in the array exactly 15 in a given array of integers.
Next: Write a C program to check if a given array of integers contains a 3 or a 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.