C Exercises: Check whether a given array of integers contains 5's and 7's
C-programming basic algorithm: Exercise-55 with Solution
Write a C program to check whether a given array of integers contains 5's and 7's.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
// Declaration and initialization of variables
int arr_size;
// Declaration and initialization of an integer array 'array1'
int array1[] = {1, 5, 6, 9, 10, 17};
// Calculating the size of the array 'array1'
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of the 'test' function for 'array1'
printf("%d",test(array1, arr_size));
// Declaration and initialization of an integer array 'array2'
int array2[] = {1, 4, 4, 9, 10, 17};
// Calculating the size of the array 'array2'
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of the 'test' function for 'array2'
printf("\n%d",test(array2, arr_size));
// Declaration and initialization of an integer array 'array3'
int array3[] = {1, 5, 5, 9, 10, 17, 5, 5};
// Calculating the size of the array 'array3'
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of the 'test' function for 'array3'
printf("\n%d",test(array3, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
// Looping through the elements of the array
for (int i = 0; i < arr_size; i++)
{
// Checking if the current element is either 5 or 7
if (nums[i] == 5 || nums[i] == 7)
{
return 1; // If condition met, return 1 (true)
}
}
return 0; // If condition not met for any element, return 0 (false)
}
Sample Output:
1 0 1
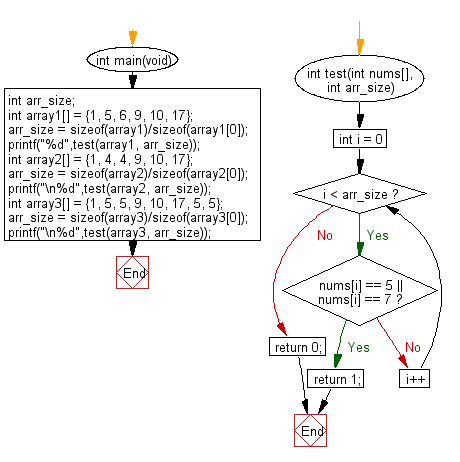
C Programming Code Editor:
Previous: Write a C program to check if a given array of integers contains 5 next to a 5 somewhere.
Next: Write a C program to check if the sum of all 5' in the array exactly 15 in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-55.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics