C Exercises: Find the largest value from first, last, and middle elements of a given array of integers of odd length
50. Largest Among Extremes
Write a C program to find the largest value from the first, last, and middle elements of a given array of integers of odd length (at least 1).
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int numbers[], int arr_size);
int main(void){
// Declaration of variables
int arr_size; // Variable to store the size of the array
// Declaration and initialization of three different arrays
int array1[] = {1};
int array2[] = {1,2,9};
int array3[] = {1,2,9,3,3};
// Calculating the size of the first array
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of 'test' function for the first array
printf("%d",test(array1, arr_size));
// Calculating the size of the second array
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of 'test' function for the second array
printf("\n%d",test(array2, arr_size));
// Calculating the size of the third array
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of 'test' function for the third array
printf("\n%d",test(array3, arr_size));
}
// Definition of the 'test' function
int test(int numbers[], int arr_size)
{
// Declaration of variables
int first, middle_ele, last_ele, max_ele;
// Initializing 'first', 'middle_ele', and 'last_ele' with values from the array
first = numbers[0];
middle_ele = numbers[arr_size / 2];
last_ele = numbers[arr_size - 1];
// Initializing 'max_ele' with the value of 'first'
max_ele = first;
// Checking if 'middle_ele' is greater than 'max_ele'
if (middle_ele > max_ele)
{
max_ele = middle_ele;
}
// Checking if 'last_ele' is greater than 'max_ele'
if (last_ele > max_ele)
{
max_ele = last_ele;
}
// Returning the maximum value
return max_ele;
}
Sample Output:
1 9 9
Pictorial Presentation:
Flowchart:
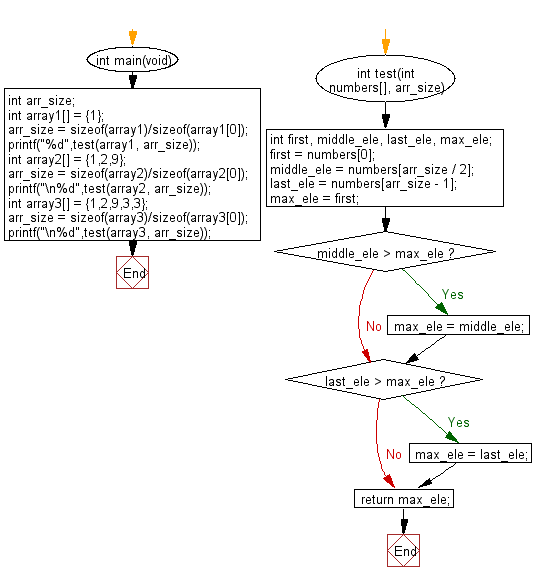
For more Practice: Solve these Related Problems:
- Write a C program to find the smallest value among the first, last, and middle elements of an odd-length array.
- Write a C program to compute the average of the first, last, and middle elements in an odd-length array.
- Write a C program to determine if the middle element of an odd-length array is greater than both the first and last elements.
- Write a C program to swap the largest of the first, middle, and last elements with the middle element.
C Programming Code Editor:
Previous: Write a C program to create a new array swapping the first and last elements of a given array of integers and length will be least 1
Next: Write a C program to count even number of elements in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.