C Exercises: Create a new array of length 3 from a given array containing the elements from the middle of the array
C-programming basic algorithm: Exercise-49 with Solution
Write a C program to create an array of length 3 from a given array (length at least 3) containing the elements from the middle of the array.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'print_array'
void print_array(int parray[], int size);
int main(void){
// Declaration of variables
int arr_size1; // Size of the original array
int nums1[] = { 1, 5, 7, 9, 11, 13 }; // Declaration and initialization of the original array 'nums1'
// Calculating the size of the original array
arr_size1 = sizeof(nums1)/sizeof(nums1[0]);
// Printing elements in the original array
printf("Elements in original array1 are: ");
print_array(nums1, arr_size1);
// Creating a new array with three elements from the middle of the original array
int result[] = { nums1[arr_size1 / 2 - 1], nums1[arr_size1 / 2], nums1[arr_size1 / 2 + 1]} ;
// Printing elements in the new array
printf("New array: ");
print_array(result, 3);
}
// Definition of the 'print_array' function
void print_array(int parray[], int size)
{
int i;
for( i=0; i<size-1; i++)
{
// Printing each element with a comma and a space
printf("%d, ", parray[i]);
}
// Printing the last element without a comma and space
printf("%d ", parray[i]);
// Printing a new line to separate the elements
printf("\n");
}
Sample Output:
Elements in original array1 are: 1, 5, 7, 9, 11, 13 New array: 7, 9, 11
Pictorial Presentation:
Flowchart:
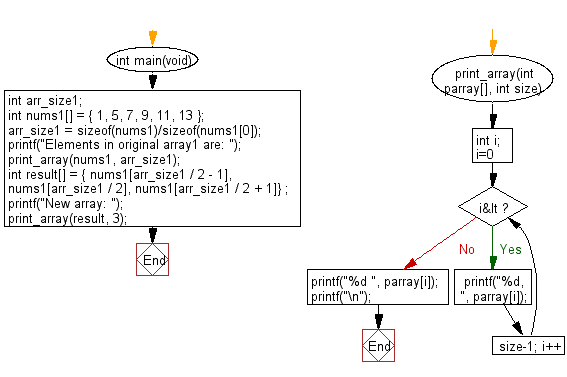
C Programming Code Editor:
Previous: Write a C program to create a new array swapping the first and last elements of a given array of integers and length will be least 1
Next: Write a C program to find the largest value from first, last, and middle elements of a given array of integers of odd length (atleast 1).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics