C Exercises: Compute the sum of the two given arrays of integers of length 3 and find the array which has the largest sum
Write a C program to compute the sum of the two given arrays of integers, length 3 and find the array that has the largest sum.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'print_array'
void print_array(int parray[], int size);
int main(void){
// Declaration and initialization of variables
int arr_size = 3;
// Declaration and initialization of arrays
int nums1[] = {10, 20, -30};
int nums2[] = {10, 20, 30};
// Printing elements in the first original array
printf("Elements in original array are: ");
print_array(nums1, arr_size);
// Printing elements in the second original array
printf("Elements in original array are: ");
print_array(nums2, arr_size);
// Declaration of result array and loop index 'i'
int result[arr_size], i;
// Comparing sums of elements in the two arrays
if (nums1[0] + nums1[1] + nums1[2] >= nums2[0] + nums2[1] + nums2[2])
{
// Copying elements from the first array to the result array
for (i = 0; i < arr_size; i++) {
result[i] = nums1[i];
}
}
else
{
// Copying elements from the second array to the result array
for (i = 0; i < arr_size; i++) {
result[i] = nums2[i];
}
}
// Printing the array with the largest sum
printf("The array which has the largest sum.: ");
print_array(result, arr_size);
}
// Definition of the 'print_array' function
void print_array(int parray[], int size)
{
int i;
for( i=0; i<size-1; i++)
{
// Printing each element with a comma and a space
printf("%d, ", parray[i]);
}
// Printing the last element without a comma and space
printf("%d ", parray[i]);
// Printing a new line to separate the elements
printf("\n");
}
Sample Output:
Elements in original array are: 10, 20, -30 Elements in original array are: 10, 20, 30 The array which has the largest sum.: 10, 20, 30
Pictorial Presentation:
Flowchart:
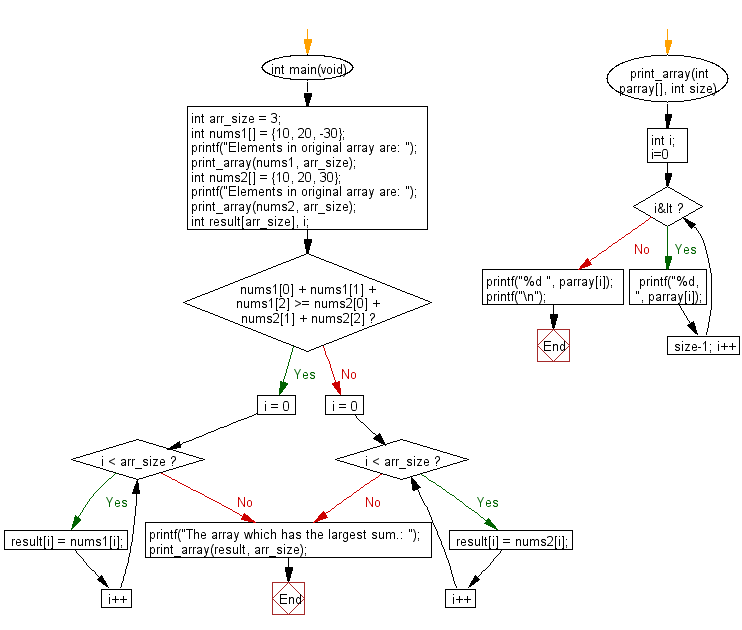
C Programming Code Editor:
Previous: Write a C program to check a given array of integers of length 3 and create a new array.If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
Next: Write a C program to create an array taking two middle elements from a given array of integers of length even.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics