C Exercises: Create a new array containing the middle elements from the two given arrays of integers, each length 5
39. Extract Middle Elements from Two Arrays
Write a C program to create a new array containing the middle elements from the two given arrays of integers, each of length 5.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'print_array'
void print_array(int parray[], int size);
int main(void){
// Declaration and initialization of variables
int arr_size;
int a1[] = {10, 20, -30, -40, 30 };
int a2[] = {10, 20, 30, 40, 30};
int arr_size1 = sizeof(a1)/sizeof(a1[0]);
int arr_size2 = sizeof(a2)/sizeof(a2[0]);
// Printing elements in the original arrays
printf("Elements in original array are:\n");
print_array(a1, arr_size1);
print_array(a2, arr_size2);
// Creating a new array with specific elements from the original arrays
int result[] = { a1[2], a2[2] };
// Calculating the size of the new array
arr_size = sizeof(result)/sizeof(result[0]);
// Printing elements in the new array
printf("Elements in new array are: ");
print_array(result, arr_size);
}
// Definition of the 'print_array' function
void print_array(int parray[], int size)
{
int i;
for( i=0; i<size-1; i++)
{
// Printing each element with a comma and a space
printf("%d, ", parray[i]);
}
// Printing the last element without a comma and space
printf("%d ", parray[i]);
// Printing a new line to separate the elements
printf("\n");
}
Sample Output:
Elements in original array are: 10, 20, -30, -40, 30 10, 20, 30, 40, 30 Elements in new array are: -30, 30
Pictorial Presentation:
Flowchart:
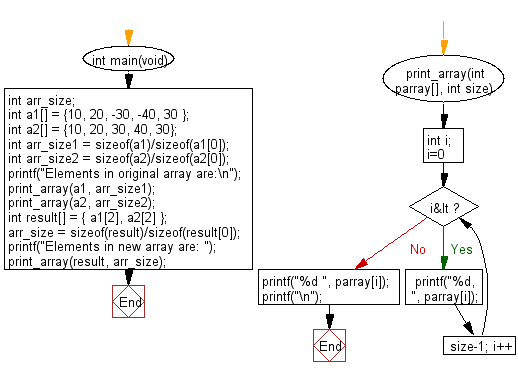
For more Practice: Solve these Related Problems:
- Write a C program to merge the middle two elements from two arrays of even length.
- Write a C program to create a new array from the middle three elements of an odd-length array.
- Write a C program to extract and swap the middle elements of two arrays of equal length.
- Write a C program to combine the middle element of one array with the first element of another.
C Programming Code Editor:
Previous: Write a C program to reverse a given array of integers and length 5.
Next: Write a C program to create a new array taking the first and last elements of a given array of integers and length 1 or more.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.