C Exercises: Compute the sum of the elements of a given array of integers
36. Sum Array Elements
Write a C program to compute the sum of the elements of an array of integers.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int a1[]);
int main(void){
// Declaration and initialization of arrays
int array1[] = {10, 20, 30, 40, 50};
int array2[] = {10, 20, -30, -40, 50};
// Printing the result of calling 'test' function with different arrays
printf("%d", test(array1));
printf("\n%d", test(array2));
}
// Definition of the 'test' function
int test(int a1[])
{
// Adding up the elements of the array and returning the result
return a1[0] + a1[1] + a1[2] + a1[3] + a1[4];
}
Sample Output:
150 10
Pictorial Presentation:
Flowchart:
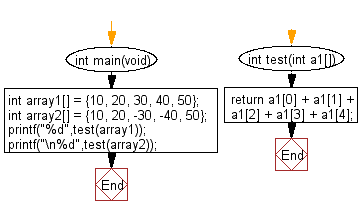
For more Practice: Solve these Related Problems:
- Write a C program to compute the product of elements in an array of integers.
- Write a C program to compute the sum of only the even numbers in an array.
- Write a C program to compute the sum of an array while ignoring negative numbers.
- Write a C program to compute the cumulative sum of an array and store it in a new array.
C Programming Code Editor:
Previous: Write a C program to check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array.
Next: Write a C program to rotate the elements of a given array of integers (length 4 ) in left direction and return the new array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.