C Exercises: Check whether a non-negative given number is a multiple of 3 or 7, but not both
Write a C program that checks if a given non-negative number is a multiple of 3 or 7, but not both.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with an integer parameter 'n'
int test(int n);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(3));
printf("\n%d",test(7));
printf("\n%d",test(21));
}
// Function definition for 'test'
int test(int n)
{
// Check if 'n' is divisible by 3 XOR divisible by 7
// XOR (^) returns true if either condition is met, but not both
if (n % 3 == 0 ^ n % 7 == 0) {
return 1; // If the XOR condition is true, return 1 (true)
} else {
return 0; // If the XOR condition is false, return 0 (false)
}
}
Sample Output:
1 1 0
Explanation:
int test(int n) { return n % 3 == 0 ^ n % 7 == 0; }
The above function takes an integer n as input and returns 1 if n is divisible by 3 or 7 but not both, otherwise it returns 0. It uses the bitwise XOR operator (^) to determine if n is divisible by only one of 3 or 7, but not both.
Time complexity and space complexity:
The time complexity of the function is O(1) as it consists of a few simple operations that do not depend on the size of the input.
The space complexity of the function is O(1) as it uses a constant amount of space regardless of the size of the input.
Pictorial Presentation:
Flowchart:
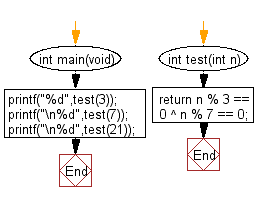
C Programming Code Editor:
Previous: Write a C program to test whether a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
Next: Write a C program to check whether a given number is within 2 of a multiple of 10.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics