C Exercises: Compute the sum of the two given integer values. If the two values are the same, then return triple their sum
Write a C program to compute the sum of the two input values. If the two values are the same, then return triple their sum.
C Code:
#include <stdio.h> // Include standard input/output library
int test(int x, int y); // Declare the function 'test' with two integer parameters
int main(void)
{
// Call the function 'test' with arguments 1 and 2 and print the result
printf("%d", test(1, 2));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 2 and 2 and print the result
printf("%d", test(2, 2));
}
// Function definition for 'test'
int test(int x, int y)
{
// Conditional expression: If x is equal to y, return (x + y) multiplied by 3, otherwise return x + y
return x == y ? (x + y) * 3 : x + y;
}
Sample Output:
3 12
Explanation:
int test(int x, int y) { return x == y ? (x + y) * 3 : x + y; }
The above function takes in two integer parameters, x and y. It uses the ternary operator to check if x is equal to y. If x is equal to y, it returns the result of (x + y)*3. Otherwise, it returns the result of x + y.
Time complexity and space complexity:
Time complexity: The time complexity of the function is O(1) as the function involves a single conditional operation and two arithmetic operations that take constant time.
Space complexity: The space complexity of the function is O(1) as the function only uses a fixed amount of space to store the variables and the return value.
Pictorial Presentation:
Flowchart:
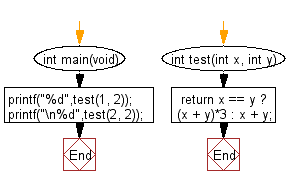
C Programming Code Editor:
Previous: C Basic Declarations and Expressions Exercises Home
Next: Write a C program to get the absolute difference between n and 51. If n is greater than 51 return triple the absolute difference.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics