C Exercises: The smallest absolute difference between X and 2 integers
Write a C program that accepts three integers: A, B, and X. Find the smallest absolute value of the difference between X and the integers between A and B.
Sample Date:
Input A, B: 7, 11
C: 20
Smallest absolute value of difference between X and integers between A and B (inclusive): 11
Input A, B: 1, 5
C: 4
Smallest absolute value of difference between X and integers between A and B (inclusive): 4
C Code:
#include <stdio.h> // Include standard input/output library
int main()
{
int A, B, X; // Declare variables for input values A, B, and X
// Prompt the user for input value A
printf("Input A: ");
// Read the input and store it in 'A'
scanf("%d", &A);
// Prompt the user for input value B
printf("Input B: ");
// Read the input and store it in 'B'
scanf("%d", &B);
// Prompt the user for input value X
printf("\nInput X: ");
// Read the input and store it in 'X'
scanf("%d", &X);
// Print a message indicating the operation being performed
printf("Smallest absolute value of difference between X and integers between A and B (inclusive):\n");
// Check conditions and print the result accordingly
if (X < A)
{
printf("%d\n", A); // Print A if X is less than A
}
else if (X > B)
{
printf("%d\n", B); // Print B if X is greater than B
}
else
{
printf("%d\n", X); // Print X if it falls between A and B
}
return 0; // Return 0 to indicate successful program execution
}
Sample Output:
Input A: 7 Input B: 11 Input X: 20 Smallest absolute value of difference between X and integers between A and B (in clusive): 11
Flowchart:
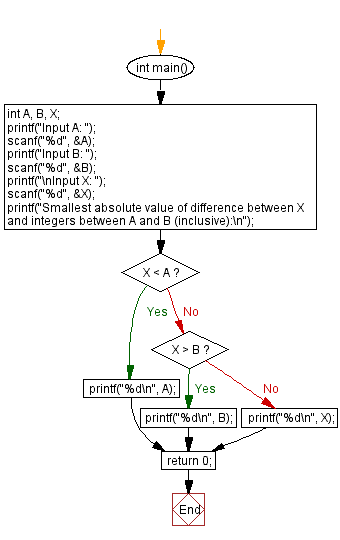
C Programming Code Editor:
Previous C Programming Exercise: Length of longest ascending contiguous subsequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics