C Exercises: Length of longest ascending contiguous subsequence
C Basic-II: Exercise-6 with Solution
Write a C program that accepts a sequence of positive integers from the user and finds the longest continuous subsequence.
Sample Date:
Length of the sequence: 5 Sequence: 5 2 3 4 1 Length of longest ascending contiguous subsequence: 5 [2 3 4] Length of the sequence: 6 Sequence: 10 20 30 40 50 60 Length of longest ascending contiguous subsequence: 6 [10 20 30 40 50 60] Length of the sequence: 3 Sequence: 5 1 3 Length of longest ascending contiguous subsequence: 2 [1 3]
C Code:
#include <stdio.h> // Include standard input/output library
int main()
{
int N; // Declare a variable 'N' to hold the number of integers
int nums[100]; // Declare an array 'nums' to store the input numbers
int i, tempp = 0, ctr = 0, max = 0; // Declare variables for iteration, counters, and maximum length
int x; // Declare a variable 'x' for temporary storage of input
// Prompt the user for the number of integers in the sequence
printf("Number of integers want to input in the first sequence: ");
// Read the input and store it in 'N'
scanf("%d", &N);
// Prompt the user to input numbers for the sequence
printf("Input the numbers:\n");
// Loop to read integers and store them in 'nums' array
for (i = 0; i< N; i++)
{
// Read an integer from the user and store it in 'nums' array
scanf("%d", &nums[i]);
}
// While loop to find the length of the longest ascending contiguous subsequence
while (tempp< (N - 1))
{
// Check if the current number is less than or equal to the next number
if (nums[tempp] <= nums[tempp + 1])
{
ctr++; // Increase counter for ascending sequence
}
else
{
// Check if the current ascending sequence is longer than the previous maximum
if (max <ctr)
max = ctr;
ctr = 0; // Reset counter for new sequence
}
tempp++; // Move to the next element
}
// Check if the last ascending sequence is longer than the previous maximum
if (max <ctr)
max = ctr;
// Print the length of the longest ascending contiguous subsequence
printf("Length of longest ascending contiguous subsequence: %d\n", max + 1);
return 0; // Return 0 to indicate successful program execution
}
Sample Output:
Number of integers want to input in the first sequence: 5 Input the numbers: 5 2 3 4 1 Length of longest ascending contiguous subsequence: 3
Flowchart:
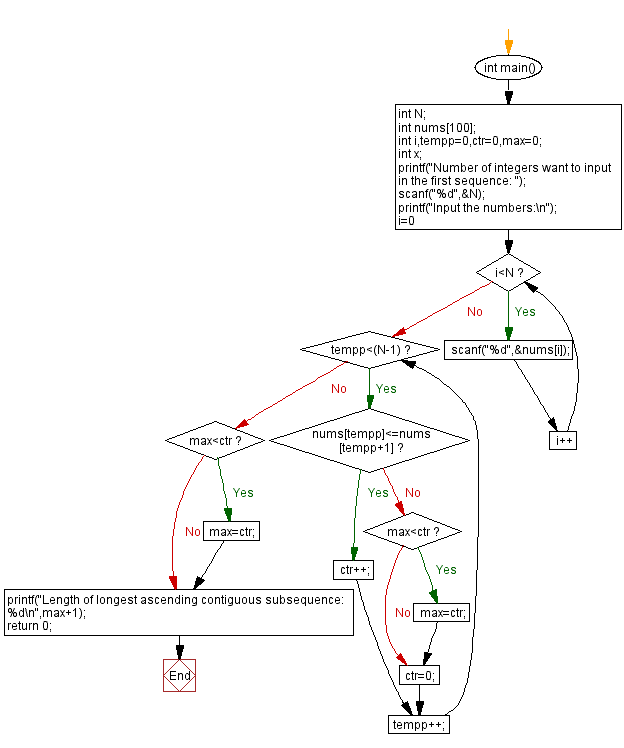
C Programming Code Editor:
Previous C Programming Exercise: Sum values before, after the maximum value in a sequence.
Next C Programming Exercise: The smallest absolute difference between X and 2 integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics