C Exercises: Second largest among three integers
Write a C program that accepts three integers from the user and finds the second largest number among them.
Constraints:
- 1≤ x ≤100
- 1≤ y ≤100
- 1≤ z ≤100
Sample Date:
(1 , 2, 3) -> 2
(10, 12, 24) -> 12
(34, 21, 30) -> 30
C Code:
#include<stdio.h> // Include standard input/output library
int test(int x, int y, int z)
{
// Function to check if 'x' is between 'y' and 'z' inclusive, or vice versa
if ((x>=y && x<=z)||(x<=y && x>=z))
return 1; // Return 1 if the condition is true
else
return 0; // Return 0 if the condition is false
}
int main()
{
int x, y, z; // Declare variables for input integers
// Prompt the user to input the first integer
printf("Input the first integer: ");
// Read the first integer from the user and store it in 'x'
scanf("%d", &x);
// Prompt the user to input the second integer
printf("Input the second integer: ");
// Read the second integer from the user and store it in 'y'
scanf("%d", &y);
// Prompt the user to input the third integer
printf("Input the third integer: ");
// Read the third integer from the user and store it in 'z'
scanf("%d", &z);
// Print a newline for formatting
printf("\n");
// Print the prompt for finding the second largest number among the three integers
printf("Second largest number among said three integers: ");
// Check conditions using the 'test' function and print the result
if (test(x,y,z))
printf("%d\n",x);
else if (test(y,x,z))
printf("%d\n",y);
else
printf("%d\n",z);
// Return 0 to indicate successful program execution
return 0;
}
Sample Output:
Input the first integer: Input the second integer: Input the third integer: 10, 12, 24 Second largest number among said three integers: 12
Flowchart:
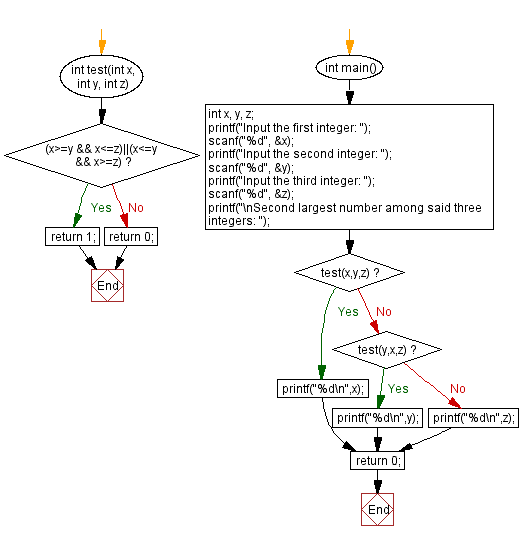
C Programming Code Editor:
Previous C Programming Exercise: Reverse a string partially.
Next C Programming Exercise: Common element(s) of two sequences.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics