C Exercises: Sort an array of 0s, 1s and 2s
54. Sort Array of 0s, 1s, and 2s
Write a program in C to sort an array of 0s, 1s and 2s.
Expected Output :
The given array is : 0 1 2 2 1 0 0 2 0 1 1 0
After sortig the elements in the array are:
0 0 0 0 0 1 1 1 1 2 2 2
To sort an array containing only 0s, 1s, and 2s, the program uses a three-way partitioning approach, such as the Dutch National Flag algorithm. This method efficiently sorts the array in a single pass by maintaining three pointers to separate the elements into three groups. The result is a sorted array with all 0s, followed by all 1s, and then all 2s.
Visual Presentation:
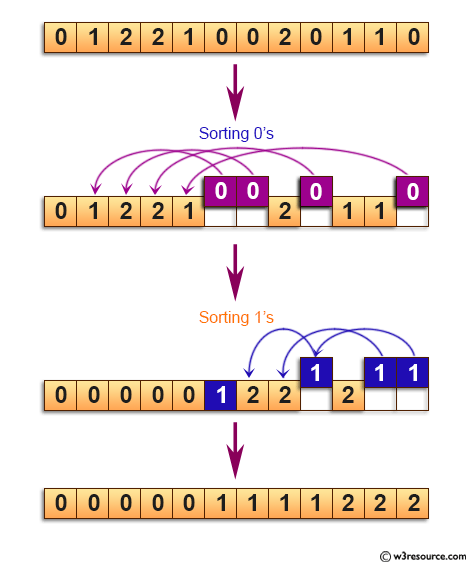
Sample Solution:
C Code:
#include <stdio.h>
// Function to swap elements at given indices i and j in the array
void changePlace(int arr1[], int i, int j) {
int tmp = arr1[i];
arr1[i] = arr1[j];
arr1[j] = tmp;
}
// Function to sort elements in the array based on a pivot value (in this case, 1)
int sortElements(int arr1[], int end) {
int start = 0, mid = 0;
int pivot = 1;
while (mid <= end) {
if (arr1[mid] < pivot) {
changePlace(arr1, start, mid);
++start, ++mid;
} else if (arr1[mid] > pivot) {
changePlace(arr1, mid, end);
--end;
} else {
++mid;
}
}
}
int main() {
int arr1[] = { 0, 1, 2, 2, 1, 0, 0, 2, 0, 1, 1, 0 };
int n = sizeof(arr1) / sizeof(arr1[0]);
int i;
//------------- print original array ------------------
printf("The given array is: ");
for (i = 0; i < n; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
//------------------------------------------------------
printf("After sorting the elements in the array are:\n");
sortElements(arr1, n - 1);
for (int i = 0 ; i < n; i++) {
printf("%d ", arr1[i]);
}
return 0;
}
Output:
The given array is : 0 1 2 2 1 0 0 2 0 1 1 0 After sortig the elements in the array are: 0 0 0 0 0 1 1 1 1 2 2 2
Flowchart:/p>
For more Practice: Solve these Related Problems:
- Write a C program to sort an array containing only 0s, 1s, and 2s using the Dutch National Flag algorithm.
- Write a C program to sort an array of 0s, 1s, and 2s without using extra space by counting frequencies first.
- Write a C program to sort an array of 0s, 1s, and 2s using recursion.
- Write a C program to sort an array of 0s, 1s, and 2s and then verify the sort by outputting counts of each element.
C Programming Code Editor:
Previous: Write a program in C to find majority element of an array.
Next: Write a program in C to check whether an array is subset of another array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.