C Exercises: Merge one sorted array into another sorted array
38. Merge Sorted Arrays
Write a program in C to merge one sorted array into another sorted array.
Note: The size of first array is (m+n) but only first m locations are populated remaining are empty. The second array is of size equal to n.
To merge one sorted array into another sorted array in C, you can implement a straightforward algorithm where you compare elements from both arrays and insert them into a new array in sorted order. This approach ensures that the resulting array remains sorted without requiring additional space beyond the arrays being merged.
.Visual Presentation:
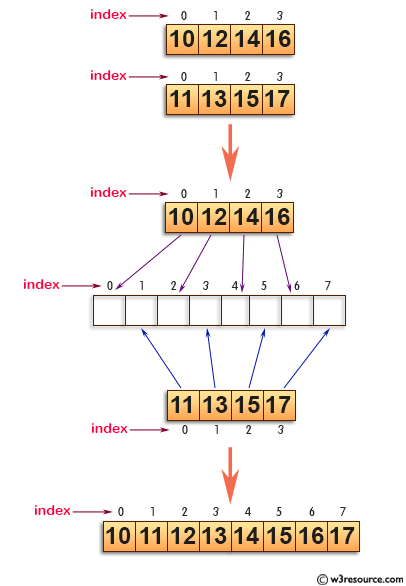
Sample Solution:
C Code:
Sample Output:
The given Large Array is : 10 12 14 16 18 20 22 The given Small Array is : 11 13 15 17 19 21 After merged the new Array is : 10 11 12 13 14 15 16 17 18 19 20 21 22
Flowchart: 1
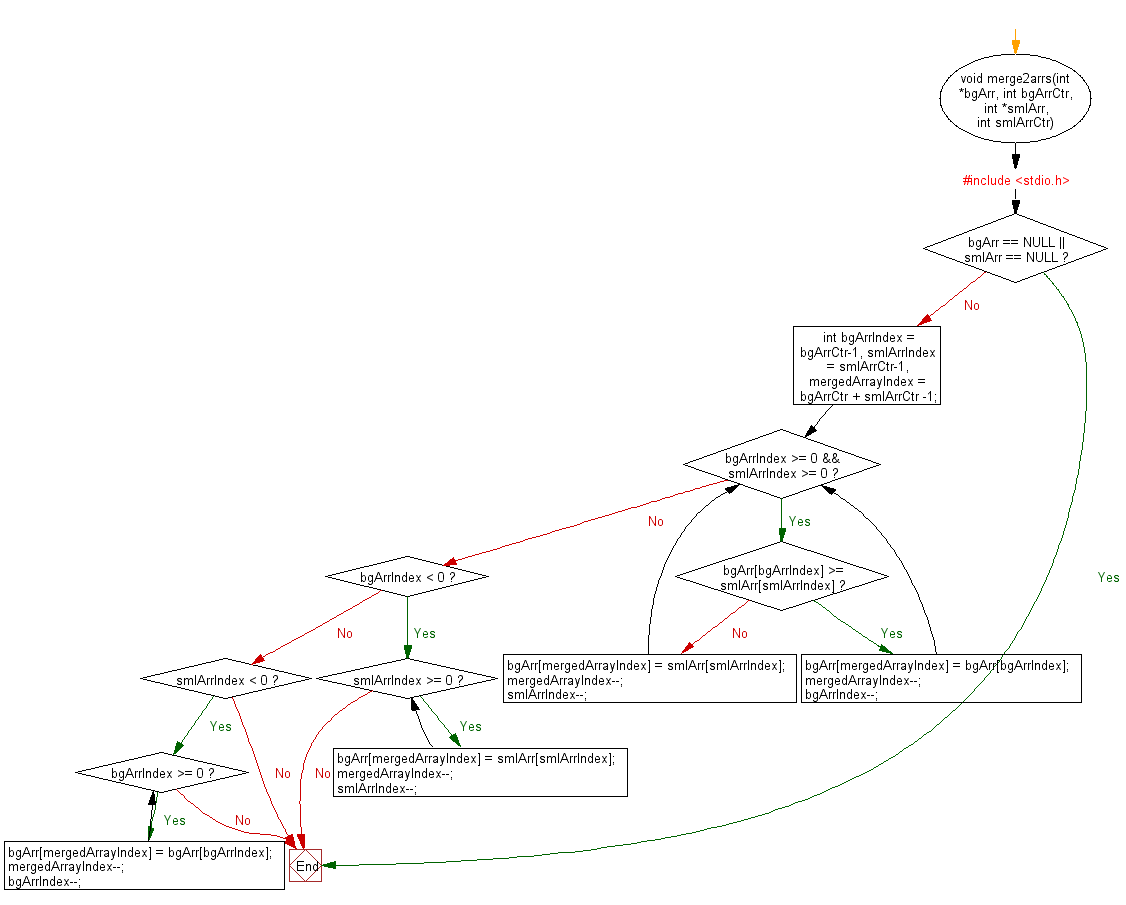
Flowchart: 2
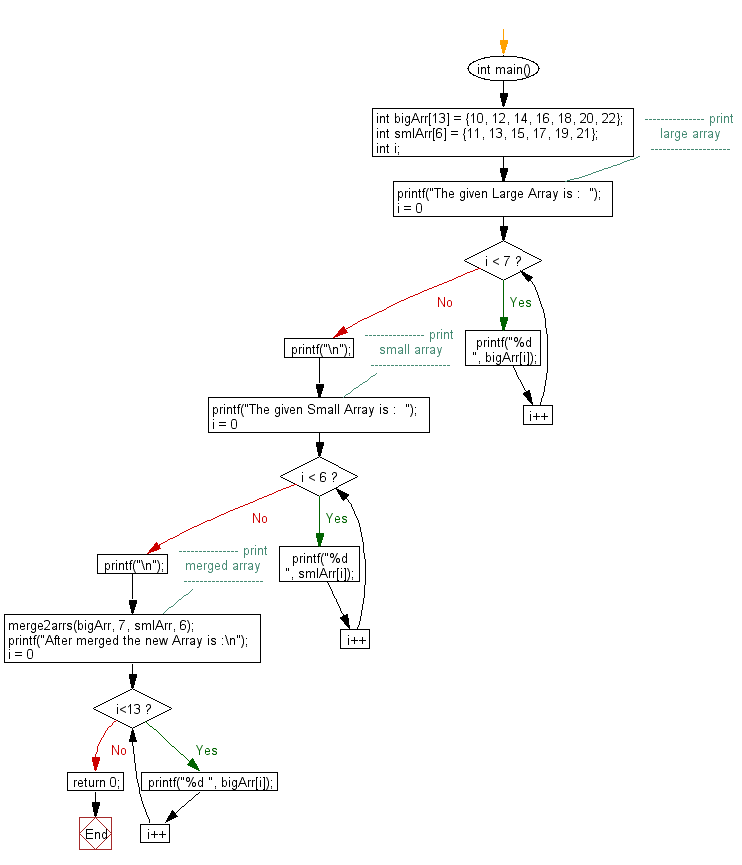
For more Practice: Solve these Related Problems:
- Write a C program to merge two sorted arrays into one sorted array using the two-pointer technique.
- Write a C program to merge two sorted arrays and remove duplicates from the merged array.
- Write a C program to merge two sorted arrays in descending order without using extra space.
- Write a C program to merge two sorted arrays and then perform a binary search on the merged array.
C Programming Code Editor:
Previous: Write a program in C to find the pivot element of a sorted and rotated array using binary search.
Next: Write a program in C to rotate an array by N positions.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.