C Exercises: Find a pair with given sum in the array
32. Pair with Given Sum
Write a program in C to find a pair with given sum in the array.
This task involves writing a C program to find a pair of elements in an array that add up to a specified sum. The program should iterate through the array, checking pairs of elements to see if their sum matches the given target. If a matching pair is found, the program outputs their indices.
Visual Presentation:
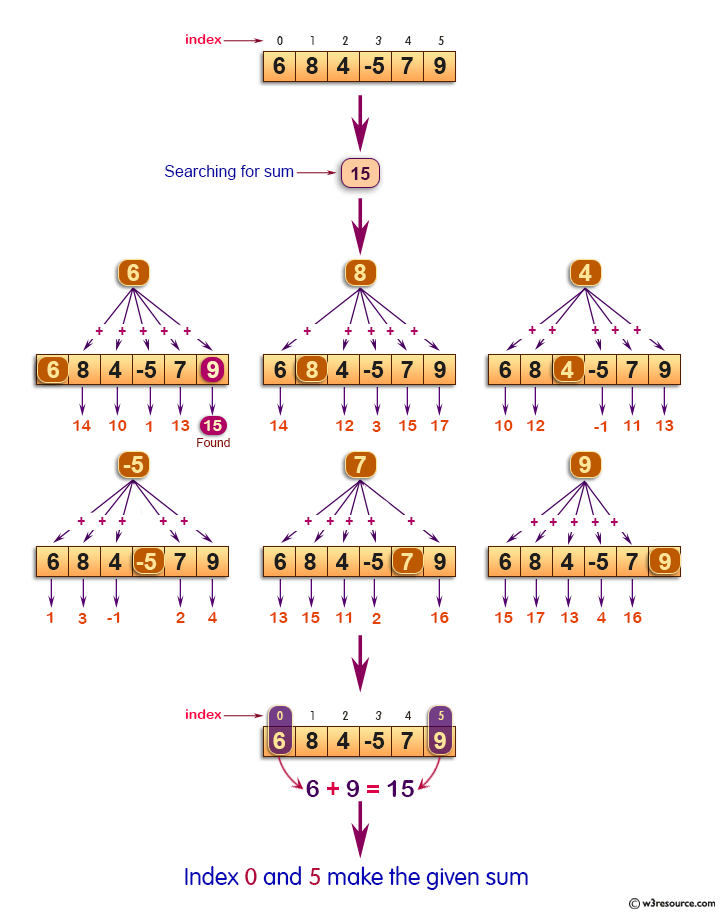
Sample Solution:
C Code:
#include <stdio.h>
// Function to check if there exists a pair of elements in the array that sums up to a given value
void checkForSum(int arr1[], int n, int s) {
// Iterate through array elements up to the second last element
for (int i = 0; i < n - 1; i++) {
// Iterate through array elements from the (i + 1)th element to the last element
for (int j = i + 1; j < n; j++) {
// Check if the sum of the current pair of elements equals the given sum
if (arr1[i] + arr1[j] == s) {
printf("Pair of elements can make the given sum by the value of index %d and %d", i, j);
return; // Exit the function as pair found
}
}
}
printf("No pair can make the given sum.");
}
// Main function
int main() {
// Array and sum initialization
int arr1[] = { 6, 8, 4, -5, 7, 9 };
int s = 15;
// Display the given array
printf("The given array : ");
int n = sizeof(arr1) / sizeof(arr1[0]);
for (int i = 0; i < n; i++) {
printf("%d ", arr1[i]);
}
printf("\nThe given sum : %d\n\n", s);
// Check for a pair of elements that sum up to the given value
checkForSum(arr1, n, s);
return 0;
}
Sample Output:
The given array : 6 8 4 -5 7 9 The given sum : 15 Pair of elements can make the given sum by the value of index 0 and 5
Flowchart :
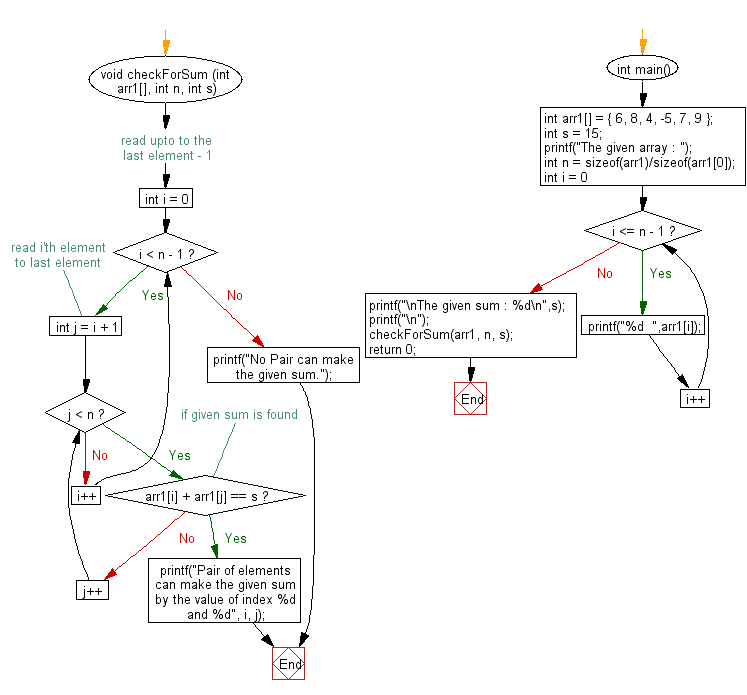
For more Practice: Solve these Related Problems:
- Write a C program to find a pair with a given sum using a hash table approach for faster lookup.
- Write a C program to identify all pairs in an array whose sum equals a specified value.
- Write a C program to find a pair with a given sum in a sorted array using two-pointer technique.
- Write a C program to find a pair with a given sum and then output the indices of the pair.
C Programming Code Editor:
Previous: Write a program in C to check whether a given matrix is an identity matrix.
Next: Write a program in C to find the majority element of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.