C Exercises: Find sum of right diagonals of a matrix
23. Right Diagonal Sum
Write a program in C to find the sum of the right diagonals of a matrix.
The task is to write a C program that calculates the sum of the elements along the right diagonal of a square matrix. The program prompts the user to input the size of the matrix and its elements, computes the sum of the elements from the top-right to bottom-left diagonal, and displays both the matrix and the sum of its right diagonal elements as output.
Visual Presentation:
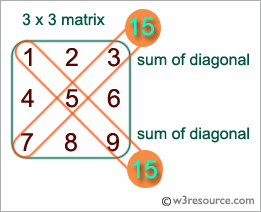
Sample Solution:
C Code:
#include <stdio.h>
int main() {
// Declare variables and matrix
int i, j, arr1[50][50], sum = 0, n;
// Display the purpose of the program
printf("\n\nFind sum of right diagonals of a matrix :\n");
printf("---------------------------------------\n");
// Input the size of the square matrix
printf("Input the size of the square matrix : ");
scanf("%d", &n);
// Input elements into the matrix and calculate sum of right diagonals
printf("Input elements in the matrix :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
// Calculate sum of elements on the right diagonal
if (i == j) {
sum = sum + arr1[i][j];
}
}
}
// Display the matrix
printf("The matrix is :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("% 4d", arr1[i][j]);
}
printf("\n");
}
// Display the sum of right diagonal elements
printf("Addition of the right Diagonal elements is : %d\n", sum);
return 0;
}
Sample Output:
Find sum of right diagonals of a matrix : --------------------------------------- Input the size of the square matrix : 2 Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [1],[0] : 3 element - [1],[1] : 4 The matrix is : 1 2 3 4 Addition of the right Diagonal elements is :5
Flowchart:
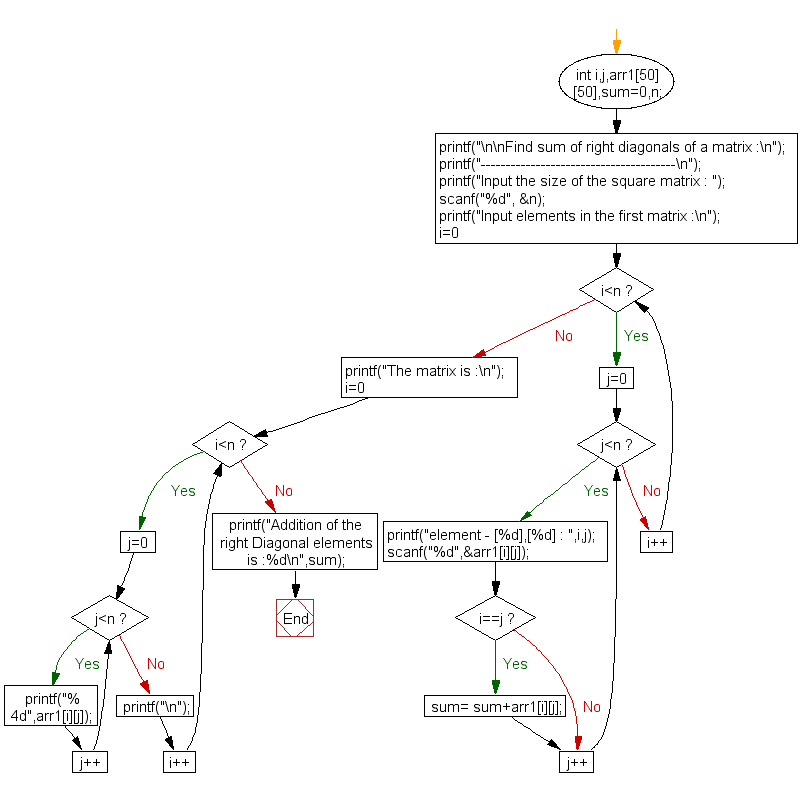
For more Practice: Solve these Related Problems:
- Write a C program to compute the sum of the primary diagonal elements of a matrix.
- Write a C program to display the elements on the right diagonal of a matrix and then compute their product.
- Write a C program to compute the right diagonal sum of a matrix using recursion.
- Write a C program to compute both the primary and secondary diagonal sums and compare them.
C Programming Code Editor:
Previous: Write a program in C to find transpose of a given matrix.
Next: Write a program in C to find sum of left diagonals of a matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.