C Exercises: Transpose of a Matrix
22. Matrix Transpose
Write a program in C to find the transpose of a given matrix.
The task is to write a C program that computes the transpose of a given matrix. The program prompts the user to input the dimensions and elements of a matrix, calculates its transpose (where rows become columns and vice versa), and then displays both the original matrix and its transpose as output.
Visual Presentation:
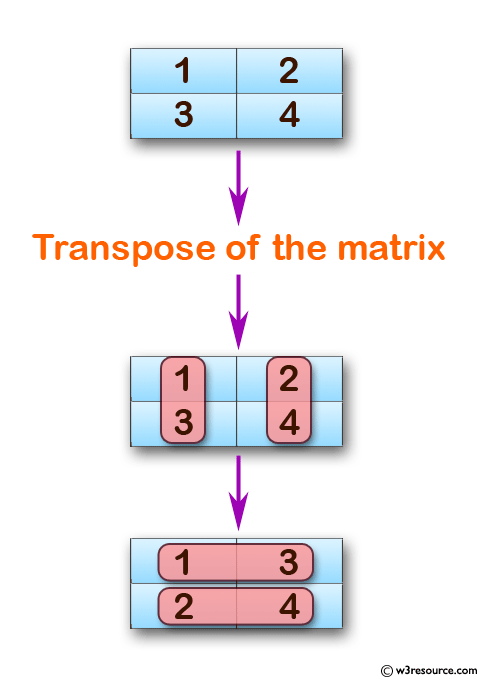
Sample Solution:
C Code:
#include <stdio.h>
int main() {
// Declare matrices and variables
int arr1[50][50], brr1[50][50], i, j, r, c;
// Display transpose of a matrix
printf("\n\nTranspose of a Matrix :\n");
printf("---------------------------\n");
// Input the rows and columns of the matrix
printf("\nInput the rows and columns of the matrix: ");
scanf("%d %d", &r, &c);
// Input elements in the first matrix
printf("Input elements in the matrix:\n");
for (i = 0; i < r; i++) {
for (j = 0; j < c; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
}
}
// Display the matrix
printf("\nThe matrix is:\n");
for (i = 0; i < r; i++) {
printf("\n");
for (j = 0; j < c; j++)
printf("%d\t", arr1[i][j]);
}
// Transpose of the matrix
for (i = 0; i < r; i++) {
for (j = 0; j < c; j++) {
// Assigning transposed values to the new matrix
brr1[j][i] = arr1[i][j];
}
}
// Display the transpose of the matrix
printf("\n\nThe transpose of a matrix is : ");
for (i = 0; i < c; i++) {
printf("\n");
for (j = 0; j < r; j++) {
printf("%d\t", brr1[i][j]);
}
}
printf("\n\n");
return 0;
}
Sample Output:
Transpose of a Matrix : --------------------------- Input the rows and columns of the matrix : 2 2 Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [1],[0] : 3 element - [1],[1] : 4 The matrix is : 1 2 3 4 The transpose of a matrix is : 1 3 2 4
Flowchart:
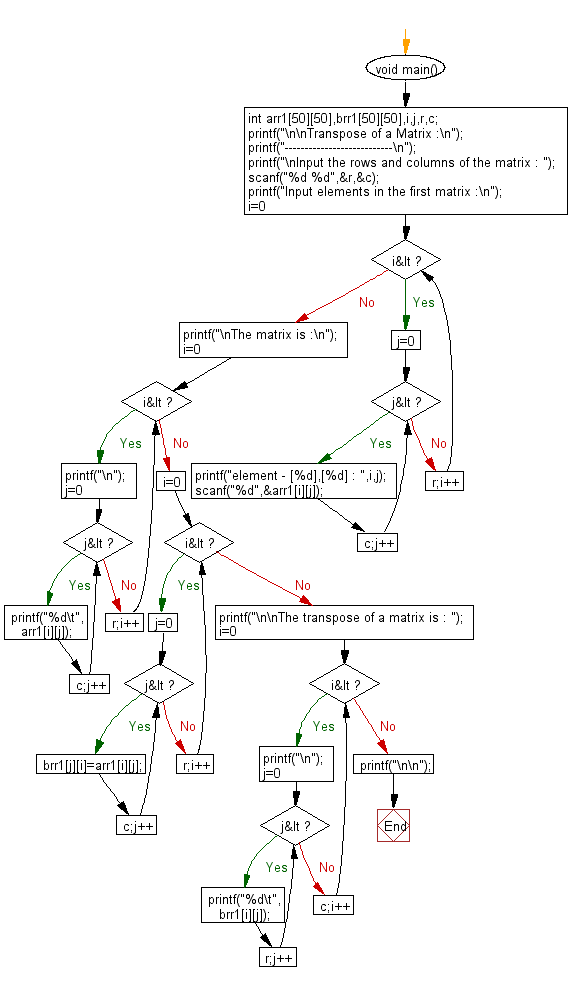
For more Practice: Solve these Related Problems:
- Write a C program to compute the transpose of a matrix and then add it to the original matrix.
- Write a C program to transpose a matrix using pointer arithmetic.
- Write a C program to compute the transpose of a matrix recursively.
- Write a C program to compute the transpose of a non-square matrix and display both matrices.
C Programming Code Editor:
Previous: Write a program in C for multiplication of two square Matrices.
Next: Write a program in C to find sum of right diagonals of a matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics