C Exercises: Read a 2D array of size 3x3 and print the matrix
18. 2D Array (3x3) & Matrix Print
Write a program in C for a 2D array of size 3x3 and print the matrix.
The task is to create a C program that defines and prints a 3x3 matrix. The program prompts the user to input elements for each position in the matrix and then displays the complete matrix as output.
Visual Presentation:
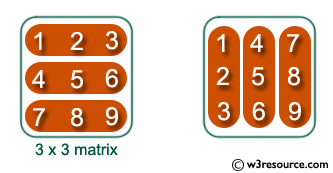
Sample Solution:
C Code:
#include <stdio.h>
void main() {
int arr1[3][3], i, j;
// Prompt user for input
printf("\n\nRead a 2D array of size 3x3 and print the matrix :\n");
printf("------------------------------------------------------\n");
// Input values for the matrix
printf("Input elements in the matrix :\n");
for (i = 0; i < 3; i++) {
for (j = 0; j < 3; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
}
}
// Display the matrix
printf("\nThe matrix is : \n");
for (i = 0; i < 3; i++) {
printf("\n");
for (j = 0; j < 3; j++)
printf("%d\t", arr1[i][j]);
}
printf("\n\n");
}
Sample Output:
Read a 2D array of size 3x3 and print the matrix : ------------------------------------------------------ Input elements in the matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [0],[2] : 3 element - [1],[0] : 4 element - [1],[1] : 5 element - [1],[2] : 6 element - [2],[0] : 7 element - [2],[1] : 8 element - [2],[2] : 9 The matrix is : 1 2 3 4 5 6 7 8 9
Flowchart:
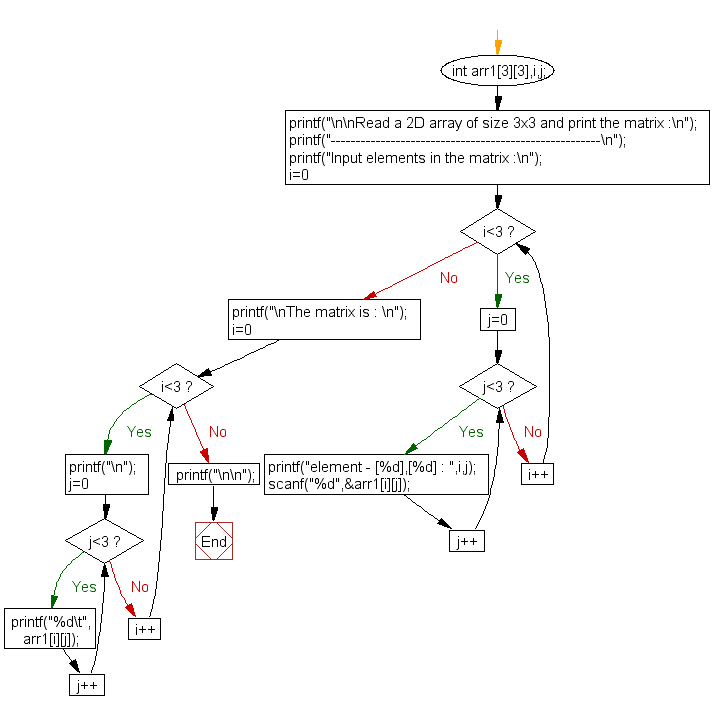
For more Practice: Solve these Related Problems:
- Write a C program to input a 3x3 matrix and then print its transpose.
- Write a C program to input a 3x3 matrix and display the matrix in spiral order.
- Write a C program to input a 3x3 matrix and compute the sum of all its elements.
- Write a C program to input a 3x3 matrix and display only the diagonal elements.
C Programming Code Editor:
Previous: Write a program in C to find the second smallest element in an array.
Next: Write a program in C for addition of two Matrices of same size.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.