C Exercises: Sort elements of array in ascending order
Write a program in C to sort elements of an array in ascending order.
Visual Presentation:
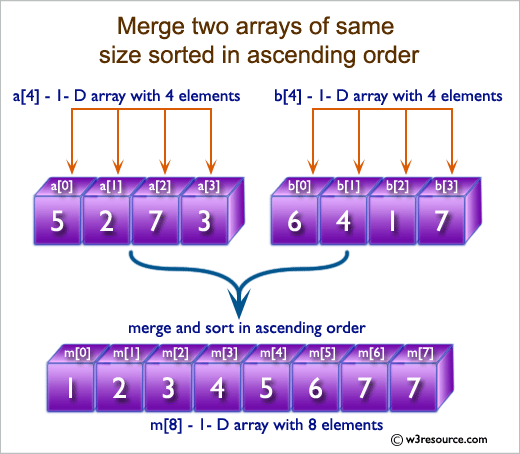
Sample Solution:
C Code:
#include <stdio.h>
void main()
{
int arr1[100];
int n, i, j, tmp;
// Prompt user for input
printf("\n\nSort elements of array in ascending order:\n");
printf("----------------------------------------------\n");
printf("Input the size of array : ");
scanf("%d", &n);
// Input elements for the array
printf("Input %d elements in the array :\n", n);
for (i = 0; i < n; i++)
{
printf("element - %d : ", i);
scanf("%d", &arr1[i]);
}
// Sorting elements in ascending order using the Bubble Sort algorithm
for (i = 0; i < n; i++)
{
for (j = i + 1; j < n; j++)
{
if (arr1[j] < arr1[i])
{
// Swap elements if they are in the wrong order
tmp = arr1[i];
arr1[i] = arr1[j];
arr1[j] = tmp;
}
}
}
// Print sorted elements in ascending order
printf("\nElements of array in sorted ascending order:\n");
for (i = 0; i < n; i++)
{
printf("%d ", arr1[i]);
}
printf("\n\n");
}
Sample Output:
sort elements of array in ascending order : ---------------------------------------------- Input the size of array : 5 Input 5 elements in the array : element - 0 : 2 element - 1 : 7 element - 2 : 4 element - 3 : 5 element - 4 : 9 Elements of array in sorted ascending order: 2 4 5 7 9
Flowchart:
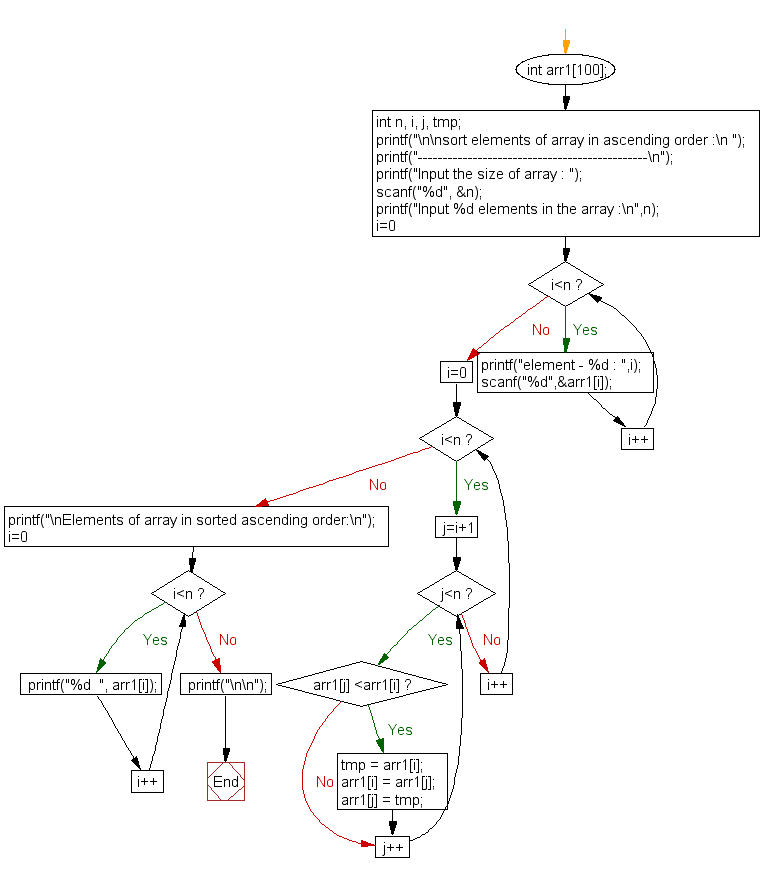
C Programming Code Editor:
Previous: Write a program in C to separate odd and even integers in separate arrays.
Next: Write a program in C to sort elements of an array in descending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics