C Exercises: Separate odd and even integers in separate arrays
10. Separate Odd & Even Arrays
Write a program in C to separate odd and even integers into separate arrays.
The task requires writing a C program to separate odd and even integers from a given array into two separate arrays. The program will take a specified number of integer inputs, store them in an array, and then create and display two new arrays: one containing all even elements and the other containing all odd elements.
Visual Presentation:
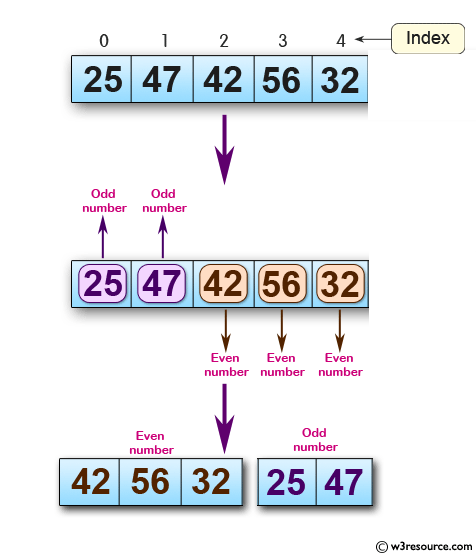
Sample Solution:
C Code:
#include <stdio.h>
int main()
{
int arr1[10], arr2[10], arr3[10];
int i, j = 0, k = 0, n;
// Prompt user for input
printf("\n\nSeparate odd and even integers in separate arrays:\n");
printf("------------------------------------------------------\n");
printf("Input the number of elements to be stored in the array :");
scanf("%d", &n);
// Input elements for the array
printf("Input %d elements in the array :\n", n);
for (i = 0; i < n; i++)
{
printf("element - %d : ", i);
scanf("%d", &arr1[i]);
}
// Separate odd and even integers into separate arrays
for (i = 0; i < n; i++)
{
if (arr1[i] % 2 == 0)
{
arr2[j] = arr1[i];
j++;
}
else
{
arr3[k] = arr1[i];
k++;
}
}
// Print the Even elements
printf("\nThe Even elements are : \n");
for (i = 0; i < j; i++)
{
printf("%d ", arr2[i]);
}
// Print the Odd elements
printf("\nThe Odd elements are :\n");
for (i = 0; i < k; i++)
{
printf("%d ", arr3[i]);
}
printf("\n\n");
return 0;
}
Sample Output:
Separate odd and even integers in separate arrays: ------------------------------------------------------ Input the number of elements to be stored in the array :5 Input 5 elements in the array : element - 0 : 25 element - 1 : 47 element - 2 : 42 element - 3 : 56 element - 4 : 32 The Even elements are : 42 56 32 The Odd elements are : 25 47
Explanation:
printf("Input the number of elements to be stored in the array :"); scanf("%d",&n); printf("Input %d elements in the array :\n",n); for(i=0;i<n;i++) { printf("element - %d : ",i); scanf("%d",&arr1[i]); } for(i=0;i<n;i++) { if (arr1[i]%2 == 0) { arr2[j] = arr1[i]; j++; } else { arr3[k] = arr1[i]; k++; } } printf("\nThe Even elements are : \n"); for(i=0;i<j;i++) { printf("%d ",arr2[i]); } printf("\nThe Odd elements are :\n"); for(i=0;i<k;i++) { printf("%d ", arr3[i]); }
In the above code -
- The first printf statement prompts the user to input the number of elements they want to store in the array and stores it in the variable n using scanf.
- The second printf statement asks the user to input n number of elements into the array arr1 using a for loop, and stores each input in the corresponding index of the array arr1[i].
- The next for loop then iterates over each element in arr1, and separates the even and odd elements into two different arrays arr2 and arr3, respectively, using if-else statements. The variables j and k are used to keep track of the indices of the even and odd elements in the two arrays.
- Finally, the last two printf statements print out the even and odd elements found, respectively, using a for loop to iterate over each element in the two arrays.
Flowchart:
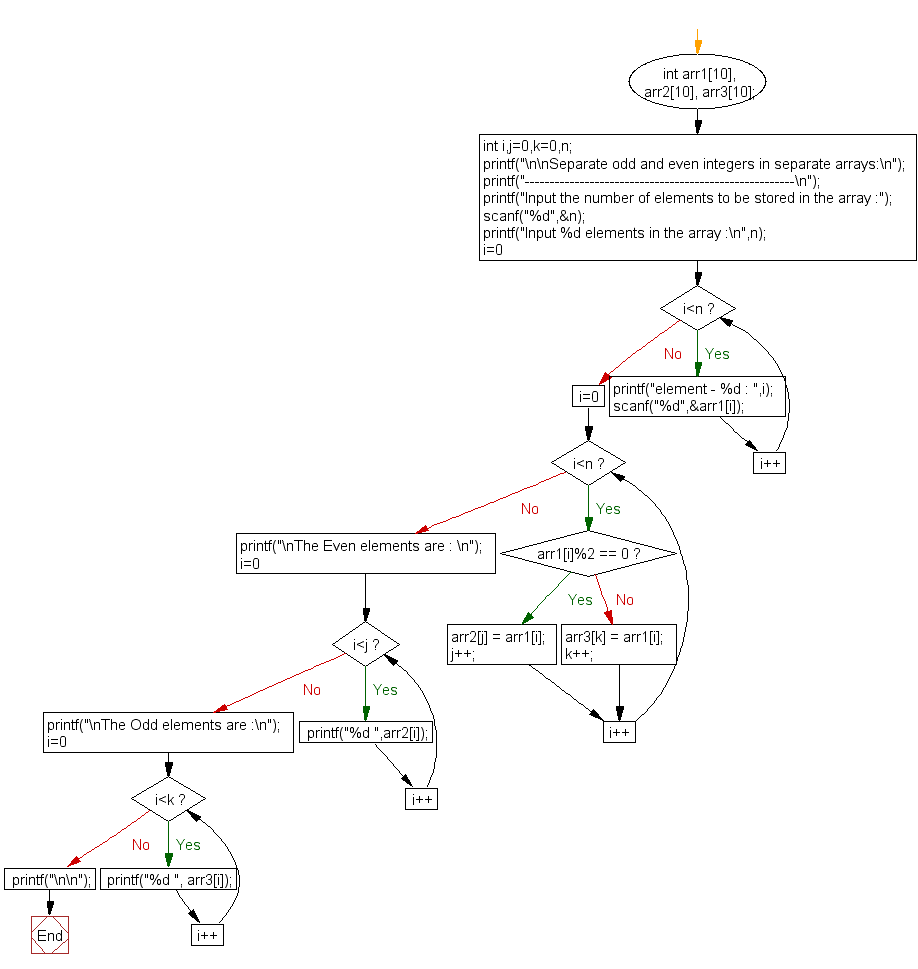
For more Practice: Solve these Related Problems:
- Write a C program to split an array into two arrays: one containing even numbers and the other containing odd numbers.
- Write a C program to separate odd and even numbers and then merge them back alternating between even and odd.
- Write a C program to count odd and even numbers in an array and then display the counts along with the separated arrays.
- Write a C program to separate odd and even numbers using pointer arithmetic and display them in sorted order.
C Programming Code Editor:
Previous: Write a program in C to find the maximum and minimum element in an array.
Next: Write a program in C to sort elements of array in ascending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.