PHP Exercises: Test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13
PHP Basic Algorithm: Exercise-41 with Solution
Write a PHP program to test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if a number satisfies a certain condition related to modulo 13
function test($n)
{
// Check if the remainder of $n divided by 13 is 0 or 1
return $n % 13 == 0 || $n % 13 == 1;
}
// Test the function with different values
var_dump(test(13));
var_dump(test(14));
var_dump(test(27));
var_dump(test(41));
?>
Explanation:
- Function Definition:
- The test function checks whether a number $n meets a specific condition involving the modulo operation with 13.
- Condition Checked:
- The function checks if $n is either:
- Divisible by 13 ($n % 13 == 0), or
- Leaves a remainder of 1 when divided by 13 ($n % 13 == 1).
- If either condition is met, it returns true; otherwise, it returns false.
Output:
bool(true) bool(true) bool(true) bool(false)
Flowchart:
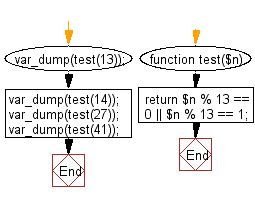
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program that accept two integers and return true if either one is 5 or their sum or difference is 5.
Next: Write a PHP program to check if a given non-negative given number is a multiple of 3 or 7, but not both.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-41.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics