C Exercises: Find the position of a target value within a sorted array using Binary search
1. Binary Search Variants
Write a C program to find the position of a target value within a sorted array using binary search.
Note: Binary Search : In computer science, a binary search or half-interval search algorithm finds the position of a target value within a sorted array. The binary search algorithm can be classified as a dichotomies divide-and-conquer search algorithm and executes in logarithmic time.
Given a sorted array arra[] of n elements, write a function to search a given element x in arra[].
Visual presentation - Binary search algorithm :
Sample Solution :
C Code :
#include<stdio.h>
void main()
{
// Variable declarations
int arra[100], i, n, x, f, l, m, flag = 0;
// Prompting user for input
printf("Input no. of elements in an array\n");
// Getting user input for the number of elements in the array
scanf("%d", &n);
// Prompting user for input
printf("Input %d value in ascending order\n", n);
// Getting user input for the elements of the array
for(i = 0; i < n; i++)
scanf("%d", &arra[i]);
// Prompting user for input
printf("Input the value to be search : ");
// Getting user input for the value to be searched
scanf("%d", &x);
// Binary Search logic
f = 0;
l = n - 1;
while (f <= l)
{
m = (f + l) / 2;
if (x == arra[m])
{
flag = 1;
break;
}
else if (x < arra[m])
l = m - 1;
else
f = m + 1;
}
// Checking if the value was found or not and printing the result
if (flag == 0)
printf("%d value not found\n", x);
else
printf("%d value found at %d position\n", x, m);
}
Sample Output:
Input no. of elements in an array 3 Input 3 value in ascending order 15 18 20 Input the value to be search : 15 15 value found at 0 position
Flowchart:
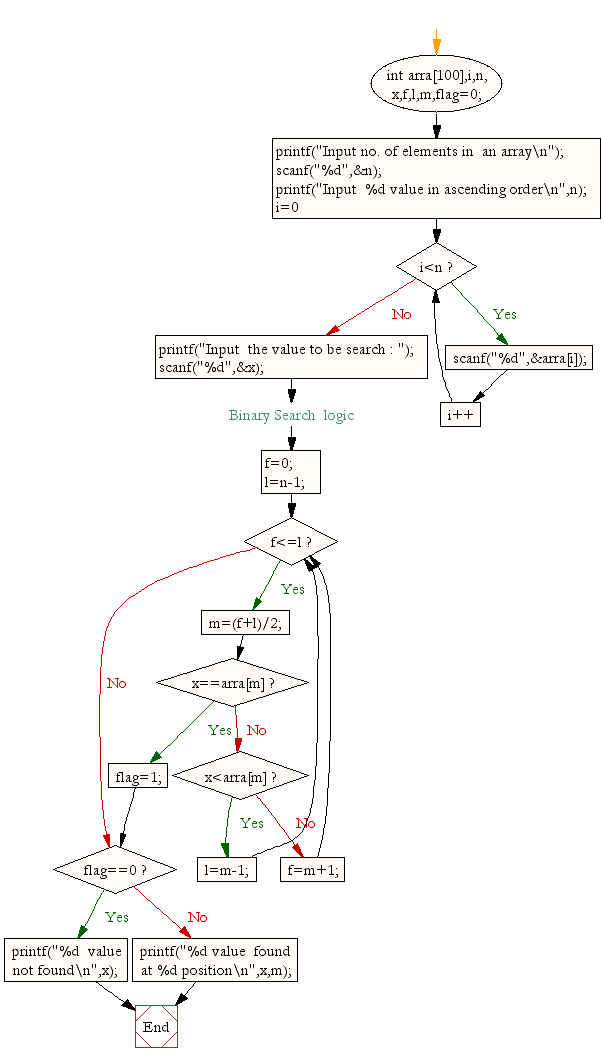
For more Practice: Solve these Related Problems:
- Write a C program to implement binary search recursively on a sorted array of integers.
- Write a C program to find the first occurrence of a target value in a sorted array with duplicate entries using binary search.
- Write a C program to perform binary search on a sorted array of strings using a custom comparator function.
- Write a C program to count the number of comparisons made during a binary search on a sorted array.
C Programming Code Editor:
Previous: C Searching and Sorting Algorithm Exercises Home
Next: Write a C program to find the position of a target value within a array using Interpolation search.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.