C Exercises: Convert a binary number into a decimal using math function
C For Loop: Exercise-46 with Solution
Write a C program to convert a binary number into a decimal number using the math function.
The task is to write a C program that converts a binary number into its decimal equivalent using mathematical functions. This involves processing each digit of the binary number and applying the appropriate power of 2 to calculate the decimal value.
Visual Presentation:
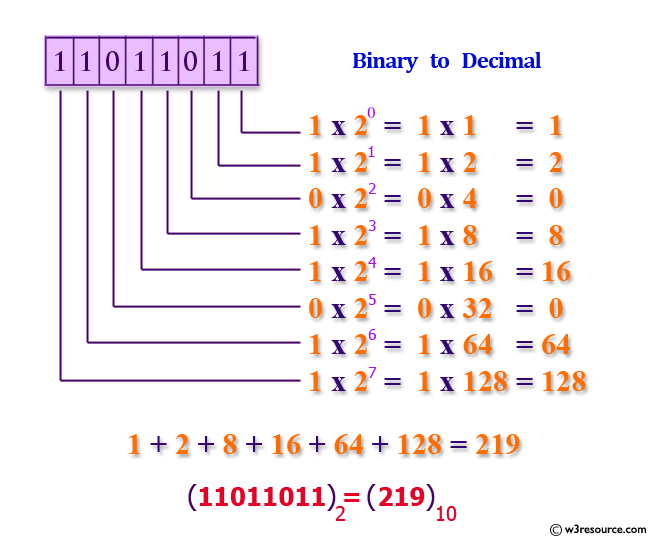
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
#include <math.h> // Include the math functions header file.
void main()
{
int n1, n; // Declare variables to store input and results.
int dec = 0, i = 0, j, d; // Initialize variables for decimal conversion.
printf("\n\nConvert Binary to Decimal:\n "); // Print a message.
printf("-------------------------\n"); // Print a separator.
printf("Input the binary number:"); // Prompt the user for input.
scanf("%d", &n); // Read the binary number from the user.
n1 = n; // Store the original binary number for display.
// Loop to convert binary to decimal.
while (n != 0)
{
d = n % 10; // Get the rightmost digit of the binary number.
dec = dec + d * pow(2, i); // Convert and accumulate the decimal value.
n = n / 10; // Remove the rightmost digit from the binary number.
i++; // Increment the position counter.
}
// Print the result.
printf("\nThe Binary Number: %d\nThe equivalent Decimal Number is: %d\n\n", n1, dec);
}
Output:
Convert Binary to Decimal: ------------------------- Input the binary number :1010100 The Binary Number : 1010100 The equivalent Decimal Number is : 84
Flowchart:
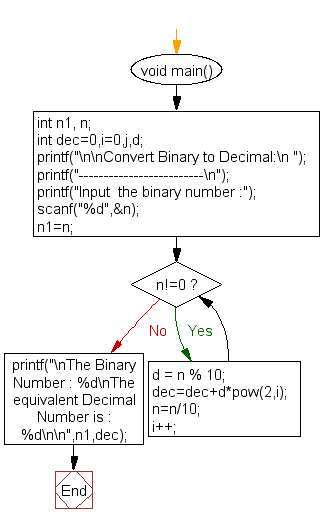
C Programming Code Editor:
Previous: Write a program in C to find LCM of any two numbers.
Next: Write a C program to check whether a number is a Strong Number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/for-loop/c-for-loop-exercises-46.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics